Messages
Messages are the main way entities communicate
amongst themselves.
They allow for greater decoupling. Entities don't have to know about each
other's capabilites or any other characteristics, they only need to know
about the types of messages.
To allow effective communication between enities, we only need to define
what a certain message type means. For this purpose the framework includes
many different and useful message types with predefined meanings.
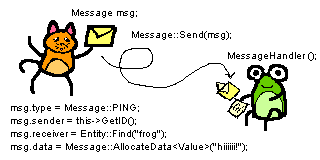
Potato cat is sending potato frog a Message.
Message types
None | NONE
Dummy message type, doesn't mean anything.
Ping | PING
Generic message type. Can be used to probe entities. When pinged, certain
entities will emit an event, or print text to console.
Move pick up | MOVE_PICK_UP
Was used to create crate stacking mechanics for immersive sims. Not used
anymore, since I added better techniques for the coordination of crate
stacking.
Open | OPEN
Used to tell doors and similar entities to open. More generally, could be
used for entities with two distinct states to assume the non-default state.
Close | CLOSE
Used to tell doors and similar entities to close. More generally, could be
used for entities with two distinct states to assume the default state.
Lock | LOCK
Used to tell doors and other entities with some kind of opening and closing
mechanism to stop opening. More generically could be used to tell enitities
to ignore certain inputs, such as the activation messages.
Unlock | UNLOCK
Similar to locking message, but in reverse.
Toggle | TOGGLE
Similar to open and close messages. Used to tell entities to toggle their
state, that is, if closed, to open, or if open, to close.
Kill | KILL
Used to tell entities to yeet themselves.
Trigger | TRIGGER
Used to pass an arbitrary message to an entity. The message data field will
then contain a pointer to a Value, usually with
the type of name.
For example, Quests from the
kitchensink extension will create a quest entity, so that they can receive
messages from Signals. Since quests have triggers,
this message is used to trigger them.
Start | START
Similar to open message, but used for entities that have some kind of
continuos action. For example, the
Sound entity uses this message to
begin playback of its associated sound.
Stop | STOP
This type is the counterpart to the start message. It tells entities to
stop their action. For example, the
Decoration entity uses this
message to stop playing its animation, if it has one.
Activate | ACTIVATE
Sent every tick from an agentic entity. Generally
meant to be used when a player presses the keyboard key associated with the
Use keyboard action while facing an entity.
Activate Once | ACTIVATE_ONCE
Similar to Activate message, but meant to be sent only for the first tick of
the activation.
Select | SELECT
Used to tell an entity that it is being selected. This could be when a
player is facing in the direction of the entity, or when the player has
placed the mouse cursor over said entity.
In response, most entities will emit a Selected
event.
Set Progress | SET_PROGRESS
Used to set the progress of the action that the entity is performing. The
data pointer of the message should be set to a Value. Usually it will be a
Float32 value in the range from 0.0f to 1.0f.
For example, the
Button entity will use
it to set its opening/closing state. A value of 0.0 will close it
completely, a value of 1.0 will open it completely and a value of 0.5 will
set it to being half-open.
Set Animation | SET_ANIMATION
Used to set the animation of an entity. The data pointer will then be set
to a Value with the type of name. The name will be the name of the
animation to which this entity should switch.
Last Mesasge | LAST_MESSAGE
Not actually a message type.
Programming in C++
#include <framework/message.h>
API documentation
page.
The API for message sending is nearly identical to that of the
Event.
Message msg;
msg.type = Message::PING;
msg.sender = this->GetID();
msg.receiver = Entity::Find("mongus")->GetID();
msg.data = Message::AllocateData<Value>(420);
// this will ping mongus
Message::Send(msg);
// we can also delay the message, pinging
// mongus 69 seconds in the future
msg.data = nullptr;
Message::Send(msg, 69.0f);
The Message::AllocateData() static method will allocate memory
for the message's data. This memory will get automatically released after
all of the messages get dispatched.
Unfortunately, due to certain limitations, this allocation works only for
messages that get sent without a delay. This will be remedied in the future.
Just like with events, we can register our own message types.
message_t type = Message::Register("frog-message");
Scripting in Lua
Essentially the same as in C++.
msg = {}
msg.type = tram.message.PING
msg.sender = 0
msg.receiver = tram.entity.Find("mongus"):GetID()
msg.data = 420
tram.message.Send(msg, 69.0)
|