Latest release
Tramway SDK 0.1.1
Github
Download
Installer (win64)
Portable .zip (win64)
Starter Template
Quick links
|
Collision Shape
The collision shape determines the volume of the of a
rigidbody or a
trigger. It consists of of a geometric shape.
Shapes
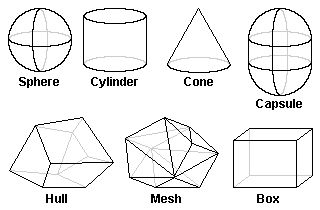
These are the shapes that you can use.
Sphere | SHAPE_SPHERE
A sphere is... well, you probably know what a sphere is. The only parameter
that it has is it's radius.
Cylinder | SHAPE_CYLINDER
A cylinder has a radius and a height.
Capsule | SHAPE_CAPSULE
A capsule is like a cylinder, but instead of having flat ends, those ends
have spheres attached to them. It has a radius and a height.
Cone | SHAPE_CONE
A cone is like a cylinder, but one of the ends has been collapsed into a
point. It has a radius and a height.
Box | SHAPE_BOX
A box is a box. It has a width, length and height. If all of these
parameters are equal, you get a cube.
Hull | SHAPE_HULL
A hull is a convex hull that is generated from a point cloud. It consists
of points, which have a position.
Mesh | SHAPE_MESH
A mesh consists of triangles. Each triangle has 3 vertices, each of which
has a position. This is shape has the worst performance, avoid it if you
can.
Programming in C++
#include <physics/collisionshape.h>
API documentation
page.
This is how you make the shapes.
const float radius = 0.5f;
const float height = 1.5f;
auto sphere = CollisionShape::Sphere(radius);
auto cylinder = CollisionShape::Cylinder(radius,
height);
auto capsule = CollisionShape::Capsule(radius,
height);
auto cone = CollisionShape::Cone(radius, height);
const vec3 dimensions = {42.0f, 6.9f, 48.9f};
auto box = CollisionShape::Box(dimensions);
const size_t cloud_size = 3;
const vec3 cloud[cloud_size] = {
{-1.0f, 1.0f, -1.0f},
{1.0f, 4.2f, 0.0f},
{-6.9f, 1.0f, -1.0f},
};
auto hull = CollisionShape::Hull(cloud, cloud_size);
const vec3 point1 = {1.0f, 1.0f, 0.0f},
const vec3 point2 = {-1.0f, 1.0f, 0.0f},
const vec3 point3 = {1.0f, -1.0f, 0.0f},
const vec3 point4 = {-1.0f, -1.0f, 0.0f},
const size_t mesh_size = 2;
CollisionTriangle triangles[mesh_size] = {
{point1, point2, point3},
{point4, point2, point3}
};
auto mesh = CollisionShape::Mesh(triangles,
mesh_size);
|















|